Quick start
Enabling code snippets
The code snippet feature can be enabled via the preferences dialog:
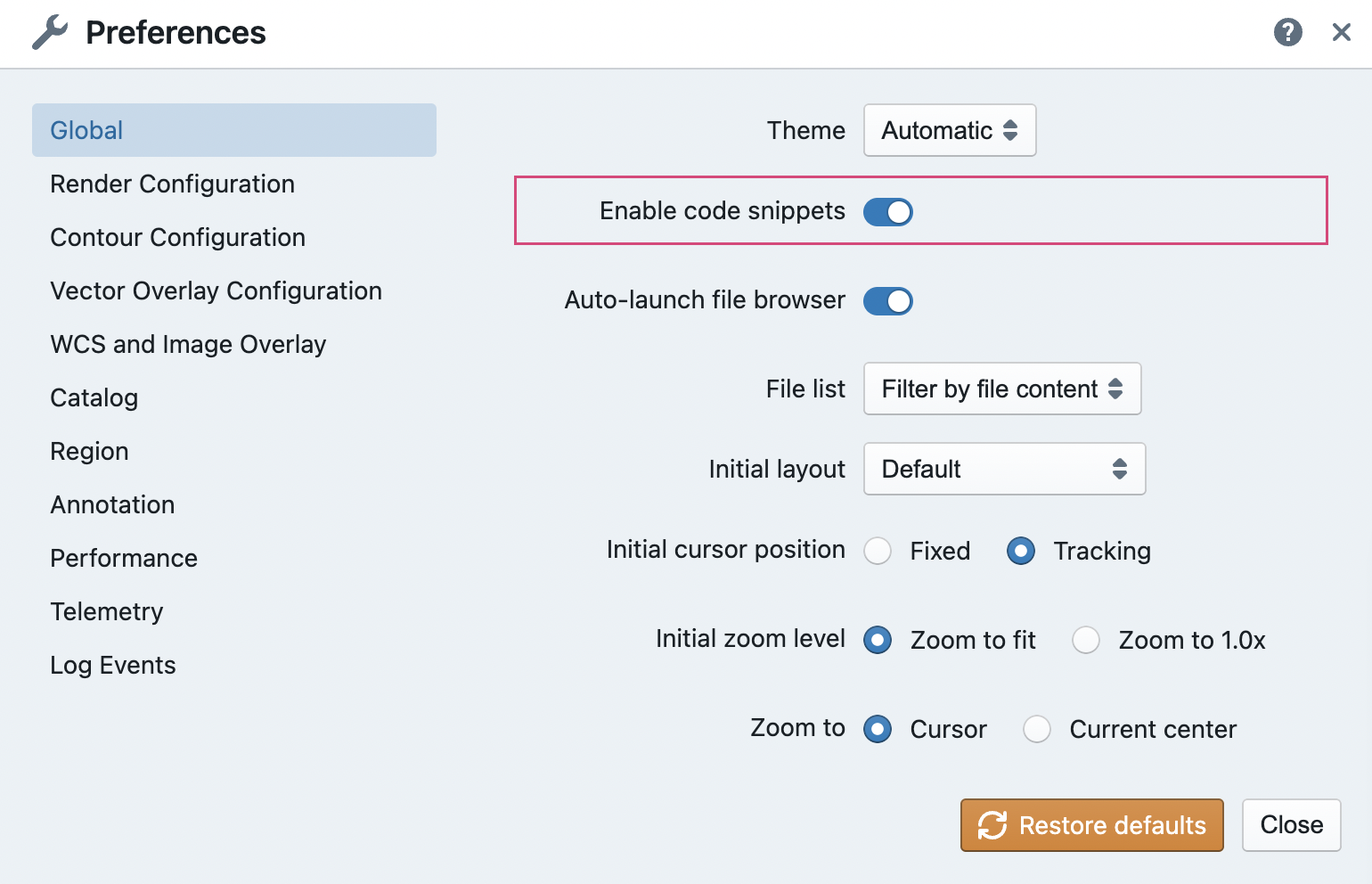
Once the code snippet feature is enabled, the "Snippets" option appears in the menu. This allows you to create and run code snippets, providing additional functionality to CARTA.
Loading images
CARTA functions and objects can be accessed via the top-level app
object (or the carta
alias). In the following example, we display the welcome splash screen for 1000 ms and then close it.
carta.showSplashScreen();
await carta.delay(1000);
app.hideSplashScreen();
Images loaded in the frontend are referred as and registered in the frames
array which contains each frame (i.e., image) as a FrameStore
object. The currently active frame is accessible with activeFrame
. In the following example, we firstly list the frames array, then list the 0th frame, and finally list the current active frame in the console.
console.log(app.frames);
console.log(app.frames[0]);
console.log(app.activeFrame);
openFile
takes up to three arguments: directory, filename and HDU. If no HDU is provided, the first HDU ("0") is adopted. The directory and filename can also be combined into a single
argument. openFile
must be called with await
, as it is an asynchronous function that requires communicating with the backend. In the following example, in the end we will see that
only the last image is loaded as each openFile
will close all loaded image first before loading the target image.
await app.openFile("test_directory", "testfile.fits", "0");
await app.openFile("test_directory", "testfile.fits");
await app.openFile("test_directory/testfile.fits");
Additional images can be appended using appendFile
. The arguments are the same as openFile
. In the following example, in the end there will be three images loaded.
const file1 = await app.openFile("testfile1.fits");
const file2 = await app.appendFile("testfile2.fits");
const file3 = await app.appendFile("testfile3.fits");
The active image can be changed with setActiveFrame
, as well as the wrapper functions setActiveFrameById
and setActiveFrameByIndex
.
app.setActiveFrameByIndex(0);
app.setActiveFrameById(file2.frameInfo.fileId);
app.setActiveFrame(file3);
Closing images
closeCurrentFile
closes the active image. There will be no user confirmation if the active image serves as the spatial reference image and there are other images matched
to it.
app.closeCurrentFile();
closeFile
takes an optional boolean argument to control whether user confirmation is required if other images are matched to the given file. This defaults to true. await
is required
to delay execution until the user confirms.
await app.closeFile(file1);
app.closeFile(file1, false); // No user confirmation
closeOtherFiles
closes all images other than the given file.
app.closeOtherFiles(file2);
For all functions and objects available in the app
object, please refer to the API documentation.